03: I/O
UC Irvine - Fall ‘22 - ICS 45C
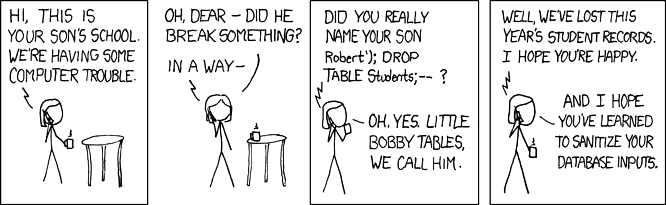
Quick list of things I want to talk about:
- Output
- cout
- using namespace std
- Input
- cin
- cout/cin multiple things
Expanded notes:
On the previous set of notes we saw how to compile a C++ code that didn’t do anything. Now, let’s try to make it say hi to you!
Output
First, let’s do the usual Hello World program. We start with the same base we had in the previous set of notes, our empty main function:
int main() {
return 0;
}
Now, in C++, we usually use cout
(stands for character output) to print things to the terminal.
But, if you try something like this:
int main() {
cout << "Hello world!\n";
return 0;
}
You’ll get an error!
cout-test.cpp:2:3: error: use of undeclared identifier 'cout'
cout << "Hello world!\n";
^
1 error generated.
This happens because cout
is not included by default.
Since some programs might not need to output things to the terminal, C++ only lets you use cout
if you include it.
It’s inside a library called iostream
.
So let’s add that:
#include <iostream>
using namespace std;
int main() {
cout << "Hello world!\n";
return 0;
}
This should work!
If you compile and run it, you should see Hello world!
printed.
Although this is a very short code, there’s actually quite a few things I want to highlight:
- We use
#include <LIBRARY>
to include a library we want, in our case it’siostream
; - The line
using namespace std;
tells C++ that we want to use the standard (std) namespace. We will cover namespaces later in the quarter, so it should make more sense later, but this line is there to let you usecout
instead of having to typestd::cout
; - To output something we use
cout << SOMETHING;
. You can imagine we are “sending” (<<
) our value to the output; - To create a string with
Hello world!
we need to use double quotes! Single quotes denote a single character in C++, so you can use'h'
but not'hi'
; - Semicolons (
;
)! Whenever you have a statement, you need to end the line with a semicolon;
Input
Okay, so we can print things now but they are pretty static. We should figure out how to read user input now.
Similar to cout
, we use cin
(character input) to read from the terminal.
cin
is also a part of iostream
, so let’s start our code like this:
#include <iostream>
using namespace std;
int main() {
return 0;
}
Now, we need somewhere to store the value.
On the next set of notes we’ll cover more variable types, but for now let’s use int
, which is the same type as our main
function.
#include <iostream>
using namespace std;
int main() {
int x;
cin >> x;
cout << x;
return 0;
}
If you compile and run this code, you should see nothing for a while, then realize that it’s waiting for you to type something… at least that’s what happened to me.
Anyway, after typing a number like 2
and pressing return, you should see the same number printed below it.
Cool! We can read things from the user now. Let’s take a look at a few things in the code:
- Before you use a variable, you need to declare it. So notice we need to do
int x;
before we can askcin
to put something in there; - To read something we use
cin >> VARIABLE;
. You can imagine we are “saving” (>>
) something to our variable; - Semicolons! More statements, more
;
throughout the code.
Multiple values
We managed to read 1 value and print 1 value. How would this work with more things?
For output, cout
will just print them one after the other.
There is no automatic line break.
If you want to add one, you can use '\n'
.
Example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello" << ' ' << 'w' << 'o' << "rld!" << '\n' << "next line!";
return 0;
}
Running this code should give you:
Hello world!
next line!
For input, cin
will try to process 1 valid value per variable.
Example:
#include <iostream>
using namespace std;
int main() {
int x;
int y;
int z;
cin >> x >> y >> z;
cout << "x=" << x << ", y=" << y << ", z=" << z << '\n';
return 0;
}
If you run this code and input:
1 2 3
You should see the output: x=1, y=2, z=3
.
It would also work with this input:
1
2
3
But if there’s some invalid input, say the string Hello
, cin
might make all values equal to zero.
References
- Comic reference: https://xkcd.com/327/